Retrieving Monitor Data using a Powershell Script
2 min read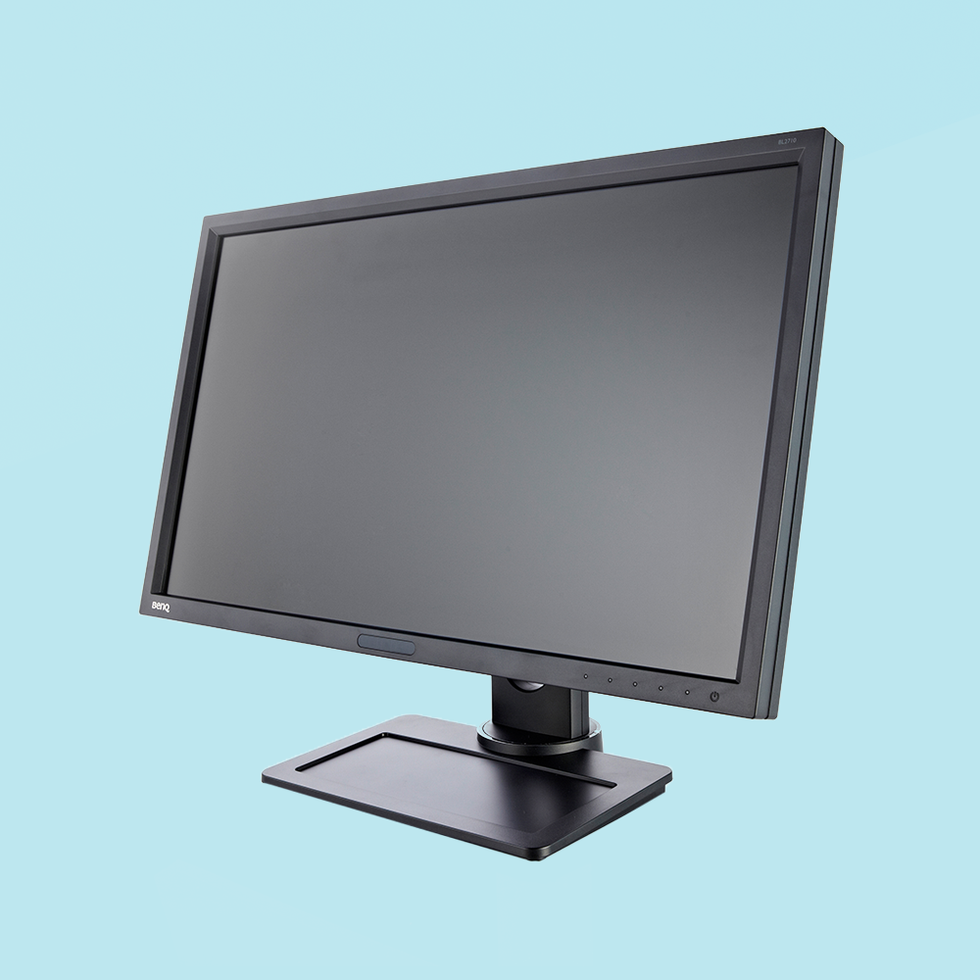
Last week I was tasked at work with figuring out the size of all our environments monitors. The monitor data being captured by SCCM was not great, and we have a lot of old monitors so my idea was to write a script and add it into the script section in SCCM and then run the script against every machine in the environment. Keep in mind if you are outputting data to a Network Drive the network drive has to have read and right access for all users because SCCM runs under the SYSTEM account and will not have access otherwise. Brief Code is below. Since SCCM Scripts rely on the machines being on at the time you run them we will run them many times. The next scripts I will write will add the data to a database and only update data if there is a change. I will create new posts when those steps come up.
This script will run a WMI query to retrieve the data and then turn the ASCII data returned to a string. I think create a Powershell object and export it to a CSV based ont he name of the computer. Then with t e second script I consolidate the data into one CSV. The reason I do this is SCCM will try to run the script simultaneously and if you try to append to a CSV it will fail if it is in use already.
Retrieves the monitor data into CSVs
$monitors = Get-WmiObject -Namespace "root\WMI" -Query "SELECT * FROM WmiMonitorID"
foreach ($monitor in $monitors) {
$edid = $monitor.UserFriendlyName -ne $null -and $monitor.UserFriendlyName.Length -gt 0
if ($edid) {
$manufacturerName = [System.Text.Encoding]::ASCII.GetString($monitor.ManufacturerName).TrimEnd([char]0x00)
$name = [System.Text.Encoding]::ASCII.GetString($monitor.UserFriendlyName).TrimEnd([char]0x00)
$serial = [System.Text.Encoding]::ASCII.GetString($monitor.SerialNumberID).TrimEnd([char]0x00)
$monitorData = [PSCustomObject]@{
ComputerName = $env:COMPUTERNAME
ManufacturerName = $manufacturerName
MonitorName = $name
SerialNumber = $serial
}
}
}
#Export to CSV Directory
$monitordata | Export-Csv "\\software\MonitorData\$env:COMPUTERNAME.csv" -NoTypeInformation -Append
Consolidate the CSVs
#Get all CSVs loaded
$CSVs = (Get-ChildItem -Path "\\software\MonitorData\").FullName
#Create array to save data from each CSV
$CSVData = @()
foreach($item in $CSVs)
{
$CSVData += Import-Csv -Path $item
}
#Export to CSV. Comment out if exporting to Database
$CSVData | Export-Csv C:\temp\MonitorExport.csv -NoTypeInformation